Coin Market
How to build an AI crypto trading bot with custom GPTs
Published
2 weeks agoon
By
AI is transforming how people interact with financial markets, and cryptocurrency trading is no exception. With tools like OpenAI’s Custom GPTs, it is now possible for beginners and enthusiasts to create intelligent trading bots capable of analyzing data, generating signals and even executing trades.
This guide analyzes the fundamentals of building a beginner-friendly AI crypto trading bot using Custom GPTs. It covers setup, strategy design, coding, testing and important considerations for safety and success.
What is a custom GPT?
A custom GPT (generative pretrained transformer) is a personalized version of OpenAI’s ChatGPT. It can be trained to follow specific instructions, work with uploaded documents and assist with niche tasks, including crypto trading bot development.
These models can help automate tedious processes, generate and troubleshoot code, analyze technical indicators and even interpret crypto news or market sentiment, making them ideal companions for building algorithmic trading bots.
What you’ll need to get started
Before creating a trading bot, the following components are necessary:
OpenAI ChatGPT Plus subscription (for access to GPT-4 and Custom GPTs).
A crypto exchange account that offers API access (e.g., Coinbase, Binance, Kraken).
Basic knowledge of Python (or willingness to learn).
A paper trading environment to safely test strategies.
Optional: A VPS or cloud server to run the bot continuously.
Did you know? Python’s creator, Guido van Rossum, named the language after Monty Python’s Flying Circus, aiming for something fun and approachable.
Step-by-step guide to building an AI trading bot with custom GPTs
Whether you’re looking to generate trade signals, interpret news sentiment or automate strategy logic, the below step-by-step approach helps you learn the basics of combining AI with crypto trading.
With sample Python scripts and output examples, you’ll see how to connect a custom GPT to a trading system, generate trade signals and automate decisions using real-time market data.
Step 1: Define a simple trading strategy
Start by identifying a basic rule-based strategy that is easy to automate. Examples include:
Buy when Bitcoin’s (BTC) daily price drops by more than 3%.
Sell when RSI (relative strength index) exceeds 70.
Enter a long position after a bullish moving average convergence divergence (MACD) crossover.
Trade based on sentiment from recent crypto headlines.
Clear, rule-based logic is essential for creating effective code and minimizing confusion for your Custom GPT.
Step 2: Create a custom GPT
To build a personalized GPT model:
Visit chat.openai.com
Navigate to Explore GPTs > Create
Name the model (e.g., “Crypto Trading Assistant”)
In the instructions section, define its role clearly. For example:
“You are a Python developer specialized in crypto trading bots.”
“You understand technical analysis and crypto APIs.”
“You help generate and debug trading bot code.”
Optional: Upload exchange API documentation or trading strategy PDFs for additional context.
Step 3: Generate the trading bot code (with GPT’s help)
Use the custom GPT to help generate a Python script. For example, type:
“Write a basic Python script that connects to Binance using ccxt and buys BTC when RSI drops below 30. I am a beginner and don’t understand code much so I need a simple and short script please.”
The GPT can provide:
Code for connecting to the exchange via API.
Technical indicator calculations using libraries like ta or TA-lib.
Trading signal logic.
Sample buy/sell execution commands.
Python libraries commonly used for such tasks are:
ccxt for multi-exchange API support.
pandas for market data manipulation.
ta or TA-Lib for technical analysis.
schedule or apscheduler for running timed tasks.
To begin, the user must install two Python libraries: ccxt for accessing the Binance API, and ta (technical analysis) for calculating the RSI. This can be done by running the following command in a terminal:
pip install ccxt ta
Next, the user should replace the placeholder API key and secret with their actual Binance API credentials. These can be generated from a Binance account dashboard. The script uses a five-minute candlestick chart to determine short-term RSI conditions.
Below is the full script:
====================================================================
import ccxt
import pandas as pd
import ta
# Your Binance API keys (use your own)
api_key = ‘YOUR_API_KEY’
api_secret = ‘YOUR_API_SECRET’
# Connect to Binance
exchange = ccxt.binance({
‘apiKey’: api_key,
‘secret’: api_secret,
‘enableRateLimit’: True,
})
# Get BTC/USDT 1h candles
bars = exchange.fetch_ohlcv(‘BTC/USDT’, timeframe=’1h’, limit=100)
df = pd.DataFrame(bars, columns=[‘timestamp’, ‘open’, ‘high’, ‘low’, ‘close’, ‘volume’])
# Calculate RSI
df[‘rsi’] = ta.momentum.RSIIndicator(df[‘close’], window=14).rsi()
# Check latest RSI value
latest_rsi = df[‘rsi’].iloc[-1]
print(f”Latest RSI: {latest_rsi}”)
# If RSI < 30, buy 0.001 BTC
if latest_rsi < 30:
order = exchange.create_market_buy_order(‘BTC/USDT’, 0.001)
print(“Buy order placed:”, order)
else:
print(“RSI not low enough to buy.”)
====================================================================
Please note that the above script is intended for illustration purposes. It does not include risk management features, error handling or safeguards against rapid trading. Beginners should test this code in a simulated environment or on Binance’s testnet before considering any use with real funds.
Also, the above code uses market orders, which execute immediately at the current price and only run once. For continuous trading, you’d put it in a loop or scheduler.
Images below show what the sample output would look like:
The sample output shows how the trading bot reacts to market conditions using the RSI indicator. When the RSI drops below 30, as seen with “Latest RSI: 27.46,” it indicates the market may be oversold, prompting the bot to place a market buy order. The order details confirm a successful trade with 0.001 BTC purchased.
If the RSI is higher, such as “41.87,” the bot prints “RSI not low enough to buy,” meaning no trade is made. This logic helps automate entry decisions, but the script has limitations like no sell condition, no continuous monitoring and no real-time risk management features, as explained previously.
Step 4: Implement risk management
Risk control is a critical component of any automated trading strategy. Ensure your bot includes:
Stop-loss and take-profit mechanisms.
Position size limits to avoid overexposure.
Rate-limiting or cooldown periods between trades.
Capital allocation controls, such as only risking 1–2% of total capital per trade.
Prompt your GPT with instructions like:
“Add a stop-loss to the RSI trading bot at 5% below the entry price.”
Step 5: Test in a paper trading environment
Never deploy untested bots with real capital. Most exchanges offer testnets or sandbox environments where trades can be simulated safely.
Alternatives include:
Running simulations on historical data (backtesting).
Logging “paper trades” to a file instead of executing real trades.
Testing ensures that logic is sound, risk is controlled and the bot performs as expected under various conditions.
Step 6: Deploy the bot for live trading (Optional)
Once the bot has passed paper trading tests:
Replace test API keys: First, replace your test API keys with live API keys from your chosen exchange’s account. These keys allow the bot to access your real trading account. To do this, log in to exchange, go to the API management section and create a new set of API keys. Copy the API key and secret into your script. It is crucial to handle these keys securely and avoid sharing them or including them in public code.
Set up secure API permissions (disable withdrawals): Adjust the security settings for your API keys. Make sure that only the permissions you need are enabled. For example, enable only “spot and margin trading” and disable permissions like “withdrawals” to reduce the risk of unauthorized fund transfers. Exchanges like Binance also allow you to limit API access to specific IP addresses, which adds another layer of protection.
Host the bot on a cloud server: If you want the bot to trade continuously without relying on your personal computer, you’ll need to host it on a cloud server. This means running the script on a virtual machine that stays online 24/7. Services like Amazon Web Services (AWS), DigitalOcean or PythonAnywhere provide this functionality. Among these, PythonAnywhere is often the easiest to set up for beginners, as it supports running Python scripts directly in a web interface.
Still, always start small and monitor the bot regularly. Mistakes or market changes can result in losses, so careful setup and ongoing supervision are essential.
Did you know? Exposed API keys are a top cause of crypto theft. Always store them in environment variables — not inside your code.
Ready-made bot templates (starter logic)
The templates below are basic strategy ideas that beginners can easily understand. They show the core logic behind when a bot should buy, like “buy when RSI is below 30.”
Even if you’re new to coding, you can take these simple ideas and ask your Custom GPT to turn them into full, working Python scripts. GPT can help you write, explain and improve the code, so you don’t need to be a developer to get started.
In addition, here is a simple checklist for building and testing a crypto trading bot using the RSI strategy:
Just choose your trading strategy, describe what you want, and let GPT do the heavy lifting, including backtesting, live trading or multi-coin support.
RSI strategy bot (buy Low RSI)
Logic: Buy BTC when RSI drops below 30 (oversold).
if rsi < 30:
place_buy_order()
Used for: Momentum reversal strategies.
Tools: ta library for RSI.
2. MACD crossover bot
Logic: Buy when MACD line crosses above signal line.
if macd > signal and previous_macd < previous_signal:
place_buy_order()
Used for: Trend-following and swing trading.
Tools: ta.trend.MACD or TA-Lib.
3. News sentiment bot
Logic: Use AI (Custom GPT) to scan headlines for bullish/bearish sentiment.
if “bullish” in sentiment_analysis(latest_headlines):
place_buy_order()
Used for: Reacting to market-moving news or tweets.
Tools: News APIs + GPT sentiment classifier.
Risks concerning AI-powered trading bots
While trading bots can be powerful tools, they also come with serious risks:
Market volatility: Sudden price swings can lead to unexpected losses.
API errors or rate limits: Improper handling can cause the bot to miss trades or place incorrect orders.
Bugs in code: A single logic error can result in repeated losses or account liquidation.
Security vulnerabilities: Storing API keys insecurely can expose your funds.
Overfitting: Bots tuned to perform well in backtests may fail in live conditions.
Always start with small amounts, use strong risk management and continuously monitor bot behavior. While AI can offer powerful support, it’s crucial to respect the risks involved. A successful trading bot combines intelligent strategy, responsible execution and ongoing learning.
Build slowly, test carefully and use your Custom GPT not just as a tool — but also as a mentor.
You may like
Coin Market
A 'local top' and $88K retest? 5 things to know in Bitcoin this week
Published
25 minutes agoon
April 28, 2025By
Bitcoin (BTC) is bracing for a major US macro data week as crypto market participants warn of serious volatility next.
Bitcoin retests $92,000 after a promising weekly close, but traders still see a deeper BTC price correction to come.
A bumper week of US macro data comes with the Federal Reserve under pressure on multiple fronts.
The Fed has its hands tied, analysis argues, predicting interest rates coming down, liquidity booming and BTC/USD reaching $180,000 within eighteen months.
Bitcoin short-term holders are back in the black, making current price levels especially pertinent for speculative investors.
Sentiment is in neutral territory, but crowd-based FOMO may keep price from rising much higher, research concludes.
Bitcoin traders wait for support retest
Bitcoin is circling multimonth highs as the week gets underway, having tested $92,000 as support after the weekly close.
BTC/USD 1-hour chart. Source: Cointelegraph/TradingView
That close itself was bullish, data from Cointelegraph Markets Pro and TradingView confirms, coming in at just above the key yearly open level of $93,500.
Can Bitcoin do it?
Can Bitcoin Weekly Close above $93500 to start the process of regaining the previous Range?$BTC #Crypto #Bitcoin https://t.co/r5reRJ0HFy pic.twitter.com/5ga0gcSqX4
— Rekt Capital (@rektcapital) April 27, 2025
Forecasting an “interesting week” to come, popular trader CrypNuevo eyed the potential for higher highs for BTC/USD.
“Pretty simple – I don’t see momentum rolling over just yet and it’s possible to see a third leg up up $97k where there is some liquidity,” he wrote in a thread on X.
“Eventually, we should see a 4H50EMA retest that can be a potential support.”BTC/USD 4-hour chart with 50 EMA. Source: Cointelegraph/TradingView
CrypNuevo referred to the 50-period exponential moving average (EMA) on 4-hour timeframes, currently at $91,850.
On the topic of likely support retests, fellow trader Roman had a deeper retracement in mind.
“Waiting to see what happens at 88k,” he told X followers.
“Not a believer in breaking 94k resistance any time soon.”BTC/USD 1-day chart with stochastic RSI data. Source: Cointelegraph/TradingView
Roman reiterated that the stochastic relative strength index (RSI) metric remained heavily overbought, a sign that a cooling-off period for price may follow.
Trader and commentator Skew meanwhile focused on the area between $90,000 and $92,000, describing “indecision” in the market resulting in current price action.
BTC/USDT 1-day chart. Source: Skew/X
GDP, PCE prints headline major macro week
It’s crunch time for US macroeconomic data and inflation progress this week, with a slew of numbers coming thick and fast.
Q1 GDP, nonfarm payrolls and tech earnings are all due, but the highlight will be the Federal Reserve’s “preferred” inflation gauge, the Personal Consumption Expenditures (PCE) index.
Set for release on April 30, both PCE and GDP precede the monthly candle close, setting the stage for crypto and risk-asset volatility.
The stakes are already high — US trade tariffs have resulted in wild swings both up and down for crypto, stocks and commodities, with seemingly no end in sight for now.
“This has been one of the most volatile years in history: The S&P 500 has seen a 2% move in either direction on 23% of trading days, or at least once a week so far this year,” trading resource The Kobeissi Letter noted in part of ongoing X analysis.
“This is the highest reading since 2022, when the share hit 29% for the full year. By comparison, the long-term average has been twice a month.”S&P 500 volatility data. Source: The Kobeissi Letter/X
Inflation expectations are a key topic, meanwhile, with markets seeing interest rate cuts beginning in June despite the Fed itself staying hawkish.
The latest data from CME Group’s FedWatch Tool shows diverging opinions over what will result from the June meeting of the Federal Open Market Committee (FOMC).
By contrast, May’s FOMC gathering is almost unanimously expected to deliver a freeze on the current Fed funds rate.
Fed target rate probabilities for June FOMC meeting. Source: CME Group
“Evidence of a strong labor market and concerns over how tariffs could impact the inflation outlook is keeping the Fed on hold when it comes to interest rates,” trading firm Mosaic Asset wrote in the latest edition of its regular newsletter, “The Market Mosaic,” on April 27.
Referencing FedWatch, Mosaic noted that “market-implied odds are starting to shift in favor of more rate cuts through year-end.”
Crypto exec doubles down on $180K BTC price target
Existing macro data is already causing a stir for crypto market participants eyeing the long-term implications of current Fed policy.
In his latest X analysis, hedge fund founder Dan Tapiero had a bold BTC price prediction in store for the coming eighteen months.
“Btc to 180k before summer ’26,” he summarized.
Tapiero pointed to a recent Fed survey showing manufacturing expectations, deteriorating at a record pace, calling the results “hard for them to ignore.”
“Forward market inflation indicators collapsing into danger zone,” he continued in a separate post on the outlook for the US Consumer Price Index (CPI).
In both cases, Tapiero concluded that Bitcoin and risk assets will benefit from increasing market liquidity — an already popular theory against the backdrop of record M2 money supply.
“Liquidity spigot coming as real rates too restrictive given fiscal tightening,” he added about current interest rates.
US CPI data. Source: Dan Tapiero/X
Bitcoin speculators turn a profit
Bitcoin short-term holders (STHs) are back under the microscope at current prices thanks to the influence of their aggregate cost basis on market trajectory.
As Cointelegraph often reports, the cost basis, also known as realized price, reflects the average price at which speculative investors entered the market.
This level, which covers buyers over the past six months but which is also broken down into various subcategories, is particularly important in Bitcoin bull markets.
“Today, when we look at the current situation, we can see that the price has reached the STH-Realized Price,” CryptoMe, a contributor to onchain analytics platform CryptoQuant, wrote in one of its “Quicktake” blog posts on the topic.
CryptoQuant shows that the combined STH cost basis currently sits at around $92,000, making the level key to hold as support going forward.
“One of the key On-Chain conditions for a bull run is that the price remains above the STH-Realized Price. If the price is below the Realized Prices, we cannot truly talk about a bull run,” CryptoMe explains.
“If this bull run is to continue, it must meet these conditions.”Bitcoin STH realized price data (screenshot). Source: CryptoQuant
The STH cost basis was lost as support in March, with the recent BTC price rebound having a near-instant impact on its most recent buyers.
STH-owned coins moving onchain earlier this month meanwhile led to predictions of fresh market volatility.
Research warns of greed-induced “local top”
After hitting its highest in nearly three months last week, greed within crypto is on the radar as a price influence this week.
Related: New Bitcoin price all-time highs could occur in May — Here is why
The latest data from the Crypto Fear & Greed Index confirms a spike to 72/100 on April 25, implying that crypto market sentiment came close to “extreme greed.”
Now back in “neutral” territory, the Index has nonetheless led research firm Santiment to warn of a potential local price top.
Crypto Fear & Greed Index (screenshot). Source: Alternative.me
“Data shows a surge in optimism from the crowd as $BTC rebounded above $95K for the first time since February,” it told X followers.
“As for the level of greed being measured across social media, this is the highest spike in bullish (vs. bearish) posts since the night Trump was elected on November 5, 2024.”Crypto market sentiment data. Source: Santiment/X
An accompanying chart covered what Santiment describes as “excitement and FOMO” peaking as a result of the BTC price rebound.
“The crowd’s level of greed vs. fear is very likely going to influence whether a local top forms (because the crowd gets too greedy), or if crypto can continue to decouple from the S&P 500 (because the crowd tries to prematurely take profit),” it added.
This article does not contain investment advice or recommendations. Every investment and trading move involves risk, and readers should conduct their own research when making a decision.
Coin Market
China-based Huawei to test AI chip aiming to rival Nvidia: Report
Published
1 hour agoon
April 28, 2025By
Chinese tech giant Huawei has reportedly developed a powerful artificial intelligence chip that could rival high-end processors from US chip maker Nvidia.
The Shenzhen-based Huawei is poised to start testing a new AI chip called the Ascend 910D, and has approached local tech firms, which are slated to receive the first batch of sample chips by late May, The Wall Street Journal reported on April 27, citing people familiar with the matter.
The development is still at an early stage, and a series of tests will be needed to assess the chip’s performance and get it ready for customers.
Huawei is pinning hopes on its latest Ascend AI processor being more powerful than Nvidia’s H100 chip, which was used for AI training in 2022.
Huawei is also poised to ship more than 800,000 earlier model Ascend 910B and 910C chips to customers, including state-owned telecoms operators and private AI developers such as TikTok parent ByteDance.
Beijing has also reportedly encouraged Chinese AI developers to increase purchases of domestic chips as trade tensions between China and the US escalate.
In mid-April, Nvidia stated that it was expecting around $5.5 billion in charges associated with its AI chip inventory due to significant export restrictions imposed by the US government affecting its business with China.
The Trump administration added Nvidia’s H20 chip, its most powerful processor that could be sold to China, to a growing list of semiconductors restricted for sale to the country.
Some key components for AI chips, such as the latest high-bandwidth memory units, have also been restricted for export to China by the US.
Huawei is focusing on building more efficient and faster systems, such as CloudMatrix 384, a computing system unveiled in April, connecting Ascend 910C chips. This would leverage their chip arrays and use brute force rather than making individual processors more powerful.
China seeks self-reliance on AI
Reuters reported on April 26, citing state media reports, that Chinese President Xi Jinping pledged “self-reliance and self-strengthening” to develop AI in the country.
“We must recognise the gaps and redouble our efforts to comprehensively advance technological innovation, industrial development, and AI-empowered applications,” Xi said at a Politburo meeting study session on April 25.
Donald Trump (left) meeting with Xi Jinping (right) in 2018 at the G20. Source: Dan Scavino
Related: US Senate bill threatens crypto, AI data centers with fees — Report
“We must continue to strengthen basic research, concentrate our efforts on mastering core technologies such as high-end chips and basic software, and build an independent, controllable, and collaborative artificial intelligence basic software and hardware system,” Xi added.
US President Donald Trump has repeatedly urged Xi to contact him for discussions about a potential trade deal after his administration imposed 145% tariffs on most Chinese goods.
China has stated that it is not having any talks with the US and that the country should “stop creating confusion.”
Magazine: Bitcoin $100K hopes on ice, SBF’s mysterious prison move: Hodler’s Digest
Coin Market
Trump-backed World Liberty Financial partners with Pakistan Crypto Council
Published
1 hour agoon
April 28, 2025By
The Donald Trump-backed World Liberty Financial has signed a Letter of Intent with the Pakistan Crypto Council to accelerate crypto adoption in the South Asian country and one of the industry’s fastest-growing markets.
Under the partnership, World Liberty will help the Council launch regulatory sandboxes to test blockchain-based products, expand stablecoin applications for remittances and trade, explore real-world asset tokenization, and assist with the growth of decentralized finance protocols, local news outlet Business Recorder reported on April 27.
World Liberty founders Zach Witkoff, Zak Folkman and Chase Herro signed the letter in a recent meeting with the Council’s CEO Bilal bin Saqib, with Pakistan’s central bank governor, finance minister and IT secretary among those in attendance.
Source: Pakistan Crypto Council
Trump and his family backed World Liberty at the crypto lending and borrowing platform’s launch last year and they receive a cut of its profits.
The Pakistan Crypto Council is a government-backed body that oversees crypto regulation and related initiatives aimed at driving adoption and attracting more foreign investment.
Blockchain analytics firm Chainalysis ranked Pakistan ninth for crypto adoption last year, with an estimated 25 million active crypto users and $300 billion in annual crypto transactions.
Pakistan is looking to capitalize on its young population, where roughly 60% are under 30, Finance Minister Muhammad Aurangzeb said.
“Pakistan’s youth and technology sector are our greatest assets. Through partnerships like this, we are opening new doors for investment, innovation, and global leadership in the blockchain economy.”
Pakistan looks to balance pro-crypto innovation with regulation
The three World Liberty founders recently met with former Binance CEO Changpeng Zhao, who was recently appointed as an adviser to the Pakistan Crypto Council to assist the country on crypto regulation and innovation.
Pakistan’s Federal Investigation Agency also proposed a crypto regulatory framework on April 10, which looks to address terrorism financing, money laundering, and Know Your Customer controls.
Related: Pakistan Crypto Council proposes using excess energy for BTC mining
FIA Director Sumera Azam said the framework is part of a broader effort to strike a “balance between technological advancement and national security imperatives.”
The proposed framework is subject to legislative approval and input from crypto firms operating in the country, with an expected multi-phased rollout beginning in 2026.
Pakistan’s new crypto-friendly approach contrasts sharply with its stance in May 2023, when former finance minister Aisha Ghaus Pasha stated the country would never legalize cryptocurrencies due to concerns over bypassing Financial Action Task Force regulations.
Magazine: Trump’s crypto ventures raise conflict of interest, insider trading questions
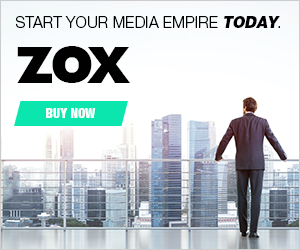

Introducing the New Carbonhand: Smarter and More Supportive Than Ever

Gunbound Rises Again – 2nd Closed Beta Goes Live for Latin America and Southeast Asia

HTX Research Latest Report | Sonic: A Model for the New DeFi Paradigm

Whiteboard Series with NEAR | Ep: 45 Joel Thorstensson from ceramic.network

New Gooseneck Omni Antennas Offer Enhanced Signals in a Durable Package

Huawei Launches Global City Intelligent Twins Architecture to Accelerate City Digital Transformation

Why You Should Build on #NEAR – Co-founder Illia Polosukhin at CV Labs

Whiteboard Series with NEAR | Ep: 45 Joel Thorstensson from ceramic.network

NEAR End of Year Town Hall 2021: The Open Web World, MetaBUILD 2 Hackathon and 2021 recap
Trending
-
Coin Market3 days ago
BlackRock, five others account for 88% of all tokenized treasury issuance
-
Coin Market2 days ago
Stripe opens testing for new stablecoin product following Bridge acquisition
-
Technology4 days ago
WASTE CONNECTIONS ANNOUNCES REGULAR QUARTERLY CASH DIVIDEND
-
Technology2 days ago
ASCP and CAP-ACP Announce Joint Annual Meeting Bringing Together U.S. and Canadian Pathologists and Medical Laboratory Professionals in 2026
-
Technology3 days ago
HitPaw Launches 2025 Global Cat Tax Campaign with Top Prizes
-
Coin Market4 days ago
Crypto users cool with AI dabbling with their portfolios: Survey
-
Technology4 days ago
J3 Selects Yardi to Manage Residential Real Estate in Kuwait
-
Technology5 days ago
PATEO Deepens Collaboration with Qualcomm to Deliver New Generation Intelligent Cockpit Solution Based on Snapdragon Cockpit Elite Platform